728x90
개념
✅ Flex: 단순한 가변 영역
✅ Grid: 복잡한 고정 영역
2차 시도
▶️ 목표: Grid로 전체 틀 잡고, 내부는 가변 성격에 따라 Flex로 구현
2-3: 완성 (Grid > Flex)
CODE
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
.recursive {
width: inherit;
height: inherit;
display: inherit;
flex-direction: inherit;
border: inherit;
}
[class*=box] {
border: solid 1px;
}
.container {
box-sizing: border-box;
width: 100%;
height: 100%;
}
.cflex {
display: flex;
flex-direction: column;
}
.rflex {
display: flex;
flex-direction: row;
}
#page {
display: grid;
width: 100%;
height: 96vh;
grid-template:
[header-left] "head nav" 1fr [header-right]
[main-left] "main main" 1fr [main-right]
[footer-left] "foot foot" 1fr [footer-right] / 3fr 2fr;
}
header {
background-color: lime;
grid-area: head;
}
nav {
background-color: lightblue;
grid-area: nav;
}
main {
background-color: yellow;
grid-area: main;
}
footer {
background-color: red;
grid-area: foot;
}
</style>
</head>
<body>
<section id="page">
<header></header>
<nav>
<div class="container cflex box">
<div class="recursive"></div>
<div class="recursive"></div>
</div>
</nav>
<main>
<div class="container rflex">
<div class="recursive cflex box">
<div class="recursive"></div>
<div class="recursive"></div>
<div class="recursive"></div>
</div>
<div class="recursive"></div>
<div class="box recursive">
<div class="recursive"></div>
<div class="recursive"></div>
<div class="recursive"></div>
</div>
</div>
</main>
<footer>
<div class="container rflex box">
<div class="recursive"></div>
<div class="recursive"></div>
<div class="recursive"></div>
<div class="recursive"></div>
<div class="recursive"></div>
</div>
</footer>
</section>
</body>
</html>
2-2: Grid로 고정 레이아웃 생성
👁️🗨️참조: grid-template - CSS: Cascading Style Sheets | MDN (mozilla.org)
✅ grid-template 하나로 종결
⭕ grid-template 스타일 하나만으로 복잡한 레이아웃 정의 가능
❌ 사전 정의된 레이아웃에서 정적인 사용 제한
CODE
더보기
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
#page {
display: grid;
width: 100%;
height: 98vh;
grid-template:
[header-left] "head nav" 1fr [header-right]
[main-left] "main main" 1fr [main-right]
[footer-left] "foot foot" 1fr [footer-right] / 3fr 2fr;
}
header {
background-color: lime;
grid-area: head;
}
nav {
background-color: lightblue;
grid-area: nav;
}
main {
background-color: yellow;
grid-area: main;
}
footer {
background-color: red;
grid-area: foot;
}
</style>
</head>
<body>
<section id="page">
<header>Header</header>
<nav>Navigation</nav>
<main>Main area</main>
<footer>Footer</footer>
</section>
</body>
</html>
2-1: Flex로 가로/세로 정렬
👁️🗨️ 스타일의 % 개념은 절대 크기가 정의된 상태에서 유효하다.
2-1-2: 재귀적 선언 및 재정의 활용
✅ OOP의 expands / @override 스타일 채용
⭕ 단순한 레이아웃 생성에 유리. 코드 단순화.
❌ 새로운 속성 추가시, CSS 선언 순서에 유의. 클래스 자체로는 content 성격 파악 불가.
CODE
더보기
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
.recursive {
width: inherit;
height: inherit;
display: inherit;
flex-direction: inherit;
border: inherit;
}
[class*=box] {
border: solid 1px;
}
body {
height: 98vh;
}
.container {
width: 100%;
height: 100%;
}
.cflex {
display: flex;
flex-direction: column;
}
.rflex {
display: flex;
flex-direction: row;
}
</style>
</head>
<body>
<div class="container rflex">
<div class="recursive cflex box">
<div class="recursive"></div>
<div class="recursive"></div>
<div class="recursive"></div>
</div>
<div class="recursive"></div>
<div class="box recursive">
<div class="recursive"></div>
<div class="recursive"></div>
<div class="recursive"></div>
</div>
</div>
</body>
</html>
2-1-1: 핵심 클래스 중심 선언
✅ 사전 정의한 형태로 레이아웃의 구조 규칙을 선언
⭕ 각각의 영역이 독립적으로 구현되어 디버깅 이점
❌ CSS 규칙 생성 및 영역 생성 시 코드 지저분
CODE
더보기
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
[class*=expand][class*=box] {
width: 100%;
height: 100%;
}
[class*=box] {
border: solid 1px;
}
body {
height: 98vh;
}
.container {
width: 100%;
height: 100%;
}
.cflex {
display: flex;
flex-direction: column;
}
.rflex {
display: flex;
flex-direction: row;
}
</style>
</head>
<body>
<div class="container rflex">
<div class="container cflex box">
<div class="box expand"></div>
<div class="box expand"></div>
<div class="box expand"></div>
</div>
<div class="container">
</div>
<div class="container rflex box">
<div class="expand box"></div>
<div class="expand box"></div>
<div class="expand box"></div>
</div>
</div>
</body>
</html>
1차 시도
더보기
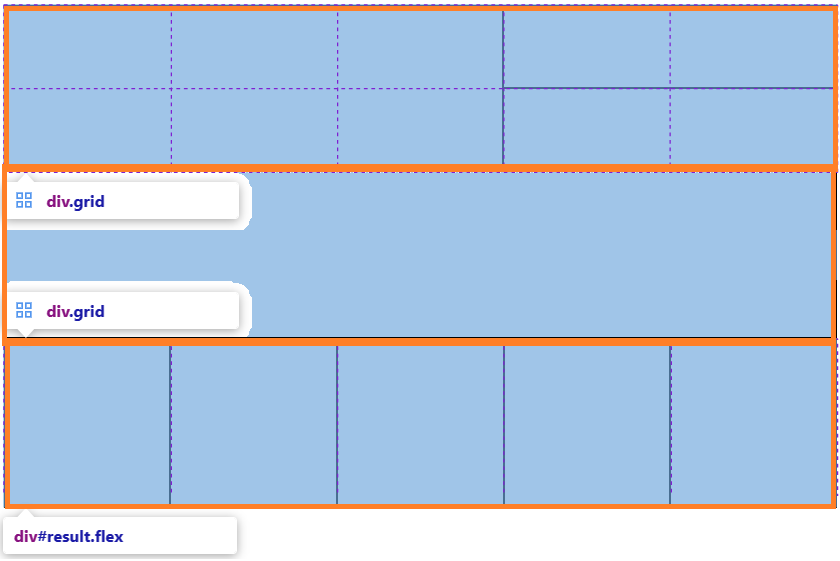
flex > grid * 3
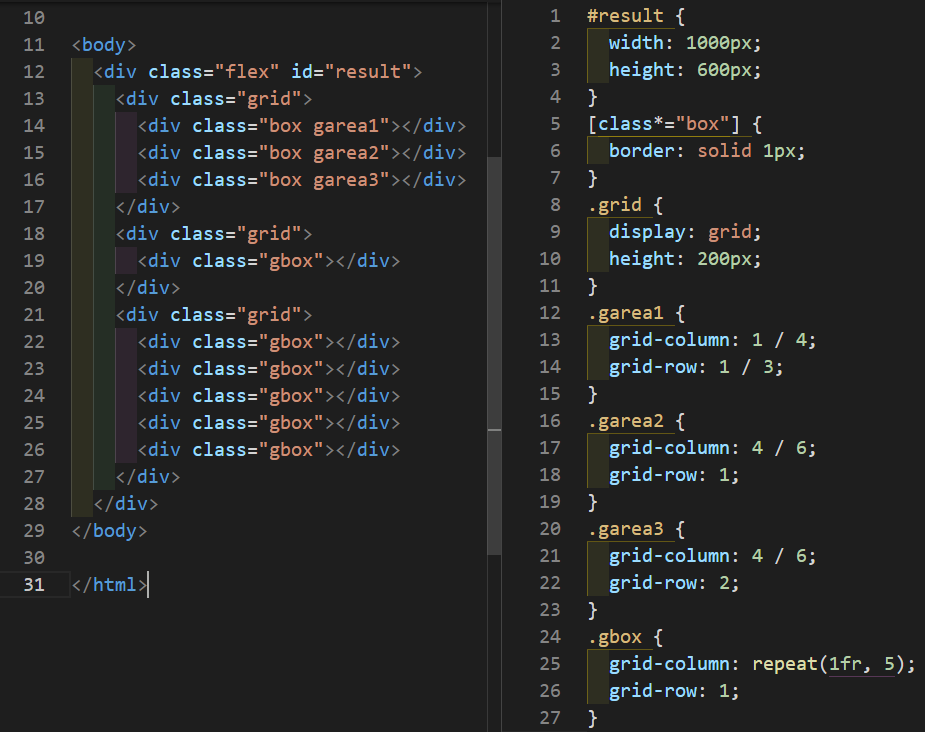
1차 시도: source image
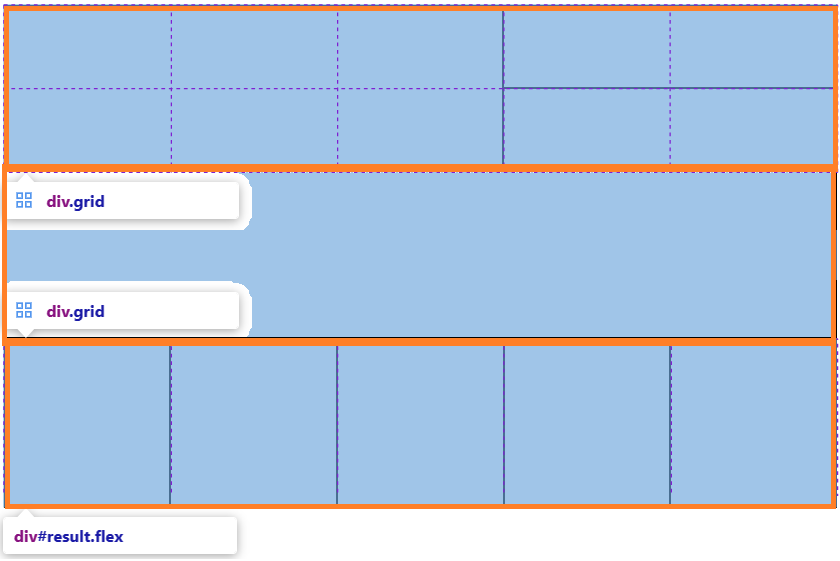
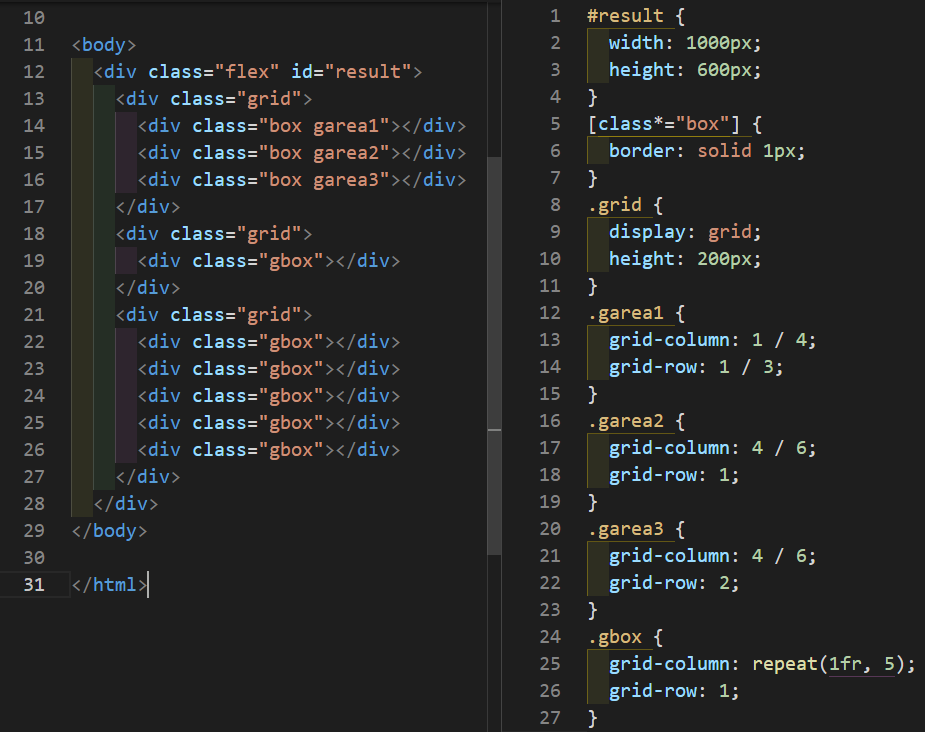
1차 시도: source text
<body>
<div class="flex" id="result">
<div class="grid">
<div class="box garea1"></div>
<div class="box garea2"></div>
<div class="box garea3"></div>
</div>
<div class="grid">
<div class="gbox"></div>
</div>
<div class="grid">
<div class="gbox"></div>
<div class="gbox"></div>
<div class="gbox"></div>
<div class="gbox"></div>
<div class="gbox"></div>
</div>
</div>
</body>
#result {
width: 1000px;
height: 600px;
}
[class*="box"] {
border: solid 1px;
}
.grid {
display: grid;
height: 200px;
}
.garea1 {
grid-column: 1 / 4;
grid-row: 1 / 3;
}
.garea2 {
grid-column: 4 / 6;
grid-row: 1;
}
.garea3 {
grid-column: 4 / 6;
grid-row: 2;
}
.gbox {
grid-column: repeat(1fr, 5);
grid-row: 1;
}
728x90
'Tip' 카테고리의 다른 글
[Python] BOJ 10815번: 숫자 카드 (0) | 2023.07.16 |
---|---|
[JavaScript] LocalStorage Basic (0) | 2023.07.13 |
[Win11] Administrator 계정 활성화 (0) | 2023.07.07 |
[Java] Singleton Pattern using synchronized (0) | 2023.07.04 |
[JavaScript] simple Counter (0) | 2023.07.03 |